I need to SVM for training data and for predicting data,having pca matrix.What is wrong in the code?It is running well but no output obtained.Neither the xml file is being saved.
#include
#include
#include "opencv2/imgcodecs.hpp"
#include
#include
using namespace cv;
using namespace cv::ml;
using namespace std;
int main()
{
Mat img_mat = imread("C:/Users/Documents//Projects/TrainingData/pic.jpg");
// Load images in the C++ format
Size size(240, 320);//the image size,e.g.64x124
resize(img_mat, img_mat, size);//resize image
int num_files = 1;//number of images
int img_area = 240 * 320;//imag size
//initialize the training matrix
//The number of rows in the matrix would be 5, and the number of columns would be the area of the image, 64*124 = 12
//Mat training_mat(num_files, img_area, CV_32FC1);
Mat training_mat(img_area, num_files, CV_32FC1);
// "fill in" the rows of training_mat with the data from each image.
cvtColor(img_mat, img_mat, CV_RGB2GRAY);
imshow("", img_mat);
int ii = 0; // Current column in training_mat
//Do this for every training image
int file_num = 0;
for (int i = 0; i(file_num, ii++) = img_mat.at(i, j);
}
}
// training matrix set up properly to pass into the SVM functions
//set up labels for each training image
//1D matrix, where each element in the 1D matrix corresponds to each row in the 2D matrix.
//-1 for non-human and 1 for human
//labels matrix
float label = 1.0;
cout << training_mat.rows << endl;
cout << training_mat.cols << endl;
//Mat labels(1, 7936, CV_32SC1, label);
Mat labels(training_mat.rows, 1, CV_32SC1, label);
//Mat labels(num_files, 1, CV_32FC1);
/*Reduce dimensionality using PCA*/
Mat projection_result;
PCA pca(training_mat, Mat(), CV_PCA_DATA_AS_ROW, 512);
pca.project(training_mat, projection_result);
/*Classification*/
// Set up SVM's parameters
Ptr svmOld = SVM::create();
svmOld->setType(SVM::C_SVC);
svmOld->setKernel(SVM::LINEAR);
svmOld->setTermCriteria(TermCriteria(TermCriteria::MAX_ITER, 100, 1e-6));
//train it based on your data
svmOld->train(training_mat, ROW_SAMPLE, labels);
//same svm
svmOld->save("svm_classification.xml");
//Initialize SVM object
Ptr svmNew = SVM::create();
//Load Previously saved SVM from XML
//can save the trained SVM so you don't have to retrain it every time
svmNew = SVM::load("svm_classification.xml");
Mat testData(1, 23040, CV_32F);
Mat test_projected;
pca.project(testData, test_projected); // should result in a 512 x 1 Mat
//int label = svmOld.predict(test_projected);
//To test your images using the trained SVM, simply read an image, convert it to a 1D matrix, and pass that in to svm
//svmNew->predict(training_mat);
//td.predict( training_mat);//It will return a value based on what you set as your labels
// to disk:
FileStorage fs("pca.xml", FileStorage::WRITE);
pca.write(fs);
fs.release(); // flush
// and back:
PCA pca;
FileStorage fs("pca.xml", FileStorage::READ);
pca.read(fs.root());
fs.release();
waitKey(0);
return(0);
}
↧
PCA+ SVM training opencv 3.0 c++
↧
Cuda Convolve VS filter2D openCV 3.1.0
Hello, I'm using OpenCV 3.1.0 with CUDA on Intel Xeon 5110 @ 1.60 Ghz x2 CPU + Nvidia Quadro 600 + 4GB RAM with Qt on Fedora 23 OS and I'm concerned about convolution speed.
What I've got from my test code is that filter2D convolution of an image with a 3x3 kernel is much faster than cuda Convolve as far as the image size is not too big (threshold around 1280x1024) and surprisingly always faster than separate convolution (first with 3x1 then 1x3 kernels), I was expecting from theory 2/3 processing time (3+3 rather than 3x3). Moreover the output image size with cuda convolve is smaller than the original one, I was expecting same size from documentation.
Is there anything wrong in what I'm doing? Any suggestion to speed up convolution for images around 640x480?
You can find below the test code I used:
cv::cuda::GpuMat temp2; // ---- is a B/W image different size
//-----fill up the temp2 image
....
//---------------------------
Mat dst_x;
Mat dst_x1;
Mat dst_x2;
Mat tmp_2;
cv::cuda::GpuMat fx;
Mat kernel_x = (Mat_(3,3) << 2, 0, -2, 4, 0, -4, 2, 0, -2);
Mat kernel_x1 = (Mat_(3,1) << 2, 4, 2); //----separate x convolution
Mat kernel_x2 = (Mat_(1,3) << 1, 0, -1);
temp2.download(tmp_2);
int64 t1 = getTickCount();
cv::filter2D(tmp_2, dst_x1, -1,kernel_x1);
cv::filter2D(dst_x1, dst_x2, -1,kernel_x2);
int64 t2 = getTick();
std::cout << "Time passed in ms: " << (((t2 - t1) / 1e9)*1000.) << std::endl;
//int64 t1 = getTickCount();
cv::filter2D(tmp_2, dst_x, -1,kernel_x);
//int64 t2 = getTick();
//std::cout << "Time passed in ms: " << (((t2 - t1) / 1e9)*1000.) << std::endl;
//----CUDA convolution---------
kernel_x.convertTo(kernel_x,CV_32FC1);
//int64 t1 = getTickCount();
Ptr convolver = cuda::createConvolution(Size(3, 3));
convolver->convolve(temp2, kernel_x, fx);
//int64 t2 = getTick();
//std::cout << "Time passed in ms: " << (((t2 - t1) / 1e9)*1000.) << std::endl;
//----END CUDA convolution---------
I can sum up the results as follows:
Image size (30,40) (rows,cols)
Time passed in ms: 0.083827filter2D convolution with kernel size (3,3)output image same size
Time passed in ms: 0.044761filter2D separated convolution with kernel size (1,3) and (3,1)output image same size
Time passed in ms: 5.95849CUDA convolve convolution with kernel size (3,3)output image size (28,38);
Image size (118,158)
Time passed in ms: 0.204968filter2D convolution with kernel size (3,3)output image same size
Time passed in ms: 0.27658filter2D separated convolution with kernel size (1,3) and (3,1)output image same size
Time passed in ms: 7.03869CUDA convolve convolution with kernel size (3,3)output image size (116,156);
Image size (469,629)
Time passed in ms: 2.51682filter2D convolution with kernel size (3,3)output image same size
Time passed in ms: 5.72645filter2D separated convolution with kernel size (1,3) and (3,1)output image same size
Time passed in ms: 9.31991CUDA convolve convolution with kernel size (3,3)output image size (467,627);
↧
↧
Opencv dnn example on Windows doesn't import caffe model properly
Hi,
I have installed Opencv3.2.0 on Windows 7 (64 bits) with dnn module using MS Visual Studio 2013 and CMake 3.7.0 as 32 bit libraries (which is needed by the main program I'm going to use dnn in).
Running the sample from [tutorial](http://docs.opencv.org/3.1.0/d5/de7/tutorial_dnn_googlenet.html) seems to read in the prototxt and binary caffemodel in,
adds the blob in with no issue, however, net.foward() crashes. I narrowed down the issue with the net.
if (net.empty())
is false, however
std::vector layernames = net.getLayerNames();
produces a vector of size 1 (instead of size 142, which is the number of layers in the bvlc_googlenet.prototxt)
I tried this all on Ubuntu 16.04 and layernames has 142 memebrs and it runs fine and produce space shuttle with 99.98% probability on that OS.
Does anyone know what the issue is on Windows and how to solve it? I also checked caffe.pb.cc and caffe.pb.h files generated by protobuf-3.1.0 (build through opencv installation) in both Windows and Ubuntu and they are the same.
Thank you
↧
ideal crop size for face recognition?
I'm using 144x144 now, but i was wondering if i should cut out the mouth and chin region, so it would not be affected so much with facial hair growth. Right my trained dataset is out of date the moment someone shaves of their beard. It's to user intrusive (and useless for any kind of monitoring to train every time this changes.
Also - would it be a good idea to segment out the face from the background on a training image? This way clothes would not be trained, and only the face.
↧
USB Camera capture is slow
Hi!
I'm trying to capture the image from my USB camera but it is slow (about 6 fps) due to the pixel format chosen by OpenCV for capturing.
My camera supports YUYV format and MJPEG format (which can provide the 30 fps) but OpenCV only use the first format.
What can I do for opening my camera with MJPG format in OpenCV ? Or should I use another method, have you an advice on which one ?
Thank you!
BTW I'm running OpenCV 3.2.0 with linux if there is a link with this problem.
↧
↧
How i can use Visual Tracker Benchmark with OpenCV
Dear community
I've found a useful dataset [Benchmark](http://cvlab.hanyang.ac.kr/tracker_benchmark/) it looks very interesting to use it to evaluate algorithm.
Actually, I use function compareHist to compare histograms using the specified method
at the end, I generate the result as a text file containing x and y coordinates of the target
I wonder if there is a possibility to test OpenCV code with this Visual Tracker Benchmark
Would be great to get your help.
↧
Gangster Hats Of The 1920'S
Whether you are a hometown cowboy or a man who prefers the look of a Western hat compared to others, then a cowboy hat is perfect for you. It's got style, but it can also help relieve the sun from your eyes. The Varmint Fur Cowboy Hat from'Hatcountry,'is classic western with its fur blend and cattle crown. It will look like you just rode away on a horse from the Lone Ranger set.
Men wore double breasted or pin striped matches or tuxedos with cutaway jackets in charcoal, navy, metallic grey or midnight red. Suits were made to gave the style of a large torso that has a cummerbund. Bow ties and also wide ties with sparkling, pressed shirts accompanied the style. Men wore fedora hats For men and frequently carried canes. Remember pictures of fashionable gangsters from your thirties? You get a perception of what men used.
![]()
Starting up her employment for a geologist, this Co Governor features constantly propagated the utilization of gas main. He or she recognized the objective as it will benefit a state around slicing wastes. The actual MOU can be a superior method of taking on marketplace limitations as compared with supplying financial aid. The fundamental assumption is always thats on sale online increased car or truck need coming from general public market should have a positive effect on buyer acquiring.
Making a dress for a grown woman would take a whole lot of time and effort, and it would likely not last very long, but there are other items to crochet that work well for adults. Beanies and other hats are easy to crochet, look fashionable, and won't come apart on you. Scarves are perhaps the simplest items to crochet, but they can be both fashionable and functional.
Beanie Baby shows are a good place to check out expensive beanies. Try to handle them if you can. Get to know the feel of the fabric and their overall appearance.
With the extracurricular load and homework leash, many students don't think they have the time for an after-school job. Too many time commitments make it impossible to juggle activities, do after school clubs and sports, complete homework, AND hold down a regular job. As a high school student, you realize that your education must come first, for if you want to make anything of yourself, a diploma is going to be beneficial. On the other hand, money is a very nice thing to have. As a student, you want to make it through school, but you'd really like to do it in fashion. The fashionable way includes the best clothes, latest gadgets, and, of course, a car to get around in. You want it all, but don't have the time to go flip burgers after a hectic day of school.
Men wore double breasted or pin striped matches or tuxedos with cutaway jackets in charcoal, navy, metallic grey or midnight red. Suits were made to gave the style of a large torso that has a cummerbund. Bow ties and also wide ties with sparkling, pressed shirts accompanied the style. Men wore fedora hats For men and frequently carried canes. Remember pictures of fashionable gangsters from your thirties? You get a perception of what men used.
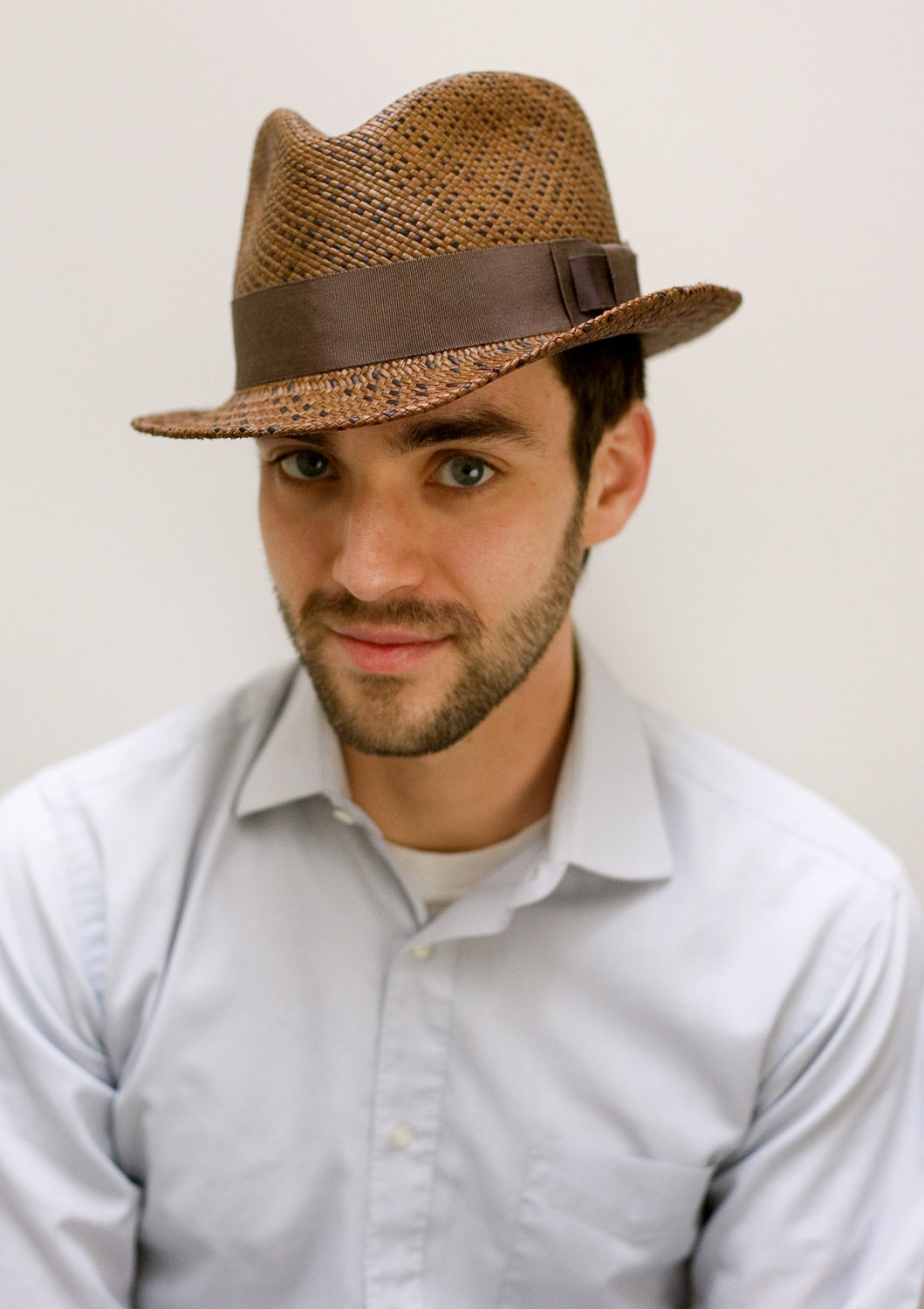
Starting up her employment for a geologist, this Co Governor features constantly propagated the utilization of gas main. He or she recognized the objective as it will benefit a state around slicing wastes. The actual MOU can be a superior method of taking on marketplace limitations as compared with supplying financial aid. The fundamental assumption is always thats on sale online increased car or truck need coming from general public market should have a positive effect on buyer acquiring.
Making a dress for a grown woman would take a whole lot of time and effort, and it would likely not last very long, but there are other items to crochet that work well for adults. Beanies and other hats are easy to crochet, look fashionable, and won't come apart on you. Scarves are perhaps the simplest items to crochet, but they can be both fashionable and functional.
Beanie Baby shows are a good place to check out expensive beanies. Try to handle them if you can. Get to know the feel of the fabric and their overall appearance.
With the extracurricular load and homework leash, many students don't think they have the time for an after-school job. Too many time commitments make it impossible to juggle activities, do after school clubs and sports, complete homework, AND hold down a regular job. As a high school student, you realize that your education must come first, for if you want to make anything of yourself, a diploma is going to be beneficial. On the other hand, money is a very nice thing to have. As a student, you want to make it through school, but you'd really like to do it in fashion. The fashionable way includes the best clothes, latest gadgets, and, of course, a car to get around in. You want it all, but don't have the time to go flip burgers after a hectic day of school.
↧
Just what to Get out of an Aircon Maintenance
You have arranged with a regional firm to have your aircon system serviced, or strategy to do so in the very near future. The trouble is you might not be exactly certain what to expect when they turn up at your residence. If you have actually never done this before, you might fidget. Fortunately is that you have hardly any to fret about as lengthy as the company you called was reputable in your community.
Introduction &Starting
When the solution crew comes to your home, it could be someone or more. Usually, you will certainly simply have a couple of people show up for a basic servicing. If issues are found that need much more substantial job, then you might have a larger crew show up to obtain the job done swiftly. For this initial meeting, expect a single person or perhaps a two individual team. Welcome them at the door equally as you would a buddy coming into your home.
Once inside, they will certainly head immediately to your aircon system. You must voice any type of problems that you have right away, so they could search for answers or look for problems if needed. You do not desire them to have to go back and inspect again after they have actually currently done their job. Obtain it full blast in the air right when they come in the door.
Clearing up Away
You can rely on a reliable solution to do their work without being kept track of. You can obtain something done around the home while they are evaluating your air conditioning system. They will involve you as well as articulate any type of concerns or fill you know anything they discover during the procedure. If everything goes efficiently and there are no problems located, then you will likely just be come close to at the end of the solution session.
Finishing the Session
As soon as the maintenance has been completed, you will be alerted of any kind of issues discovered with the system. You will certainly be educated of what has to be done following and may be advised on a period for having additional examinations or various other maintenance tasks for your system. It is very important to take notice of exactly what is being said as it might affect solutions that you may spend for in the future.
Hear all referrals from the service group, yet bear in mind that you do not need to accept any type of fixings or future solutions. If you rely on the service and also know that they have a reputation for being truthful, after that you need to probably just follow their recommendations. If they caution that a part requires changed, it is critical that you proceed and also change it. Once you have to transform the system on and start utilizing it throughout hot weather, the provider will be extremely busy with repair phone calls as well as might take longer to come out as well as fix your system.
If you are worried about the cost of replacement parts, take into consideration the expense of a completely system damage down on a warm summer day. In many cases, you are getting off affordable by just changing the component when it wases initially located to be looking for substitute. You save lots of time too, considering that you do not have to arrange a rush repair work when the part lastly provides completely.
When you have any kind of inquiries about exactly where and how to utilize aircon service, it is possible to contact us in our own internet site.

When the solution crew comes to your home, it could be someone or more. Usually, you will certainly simply have a couple of people show up for a basic servicing. If issues are found that need much more substantial job, then you might have a larger crew show up to obtain the job done swiftly. For this initial meeting, expect a single person or perhaps a two individual team. Welcome them at the door equally as you would a buddy coming into your home.
Once inside, they will certainly head immediately to your aircon system. You must voice any type of problems that you have right away, so they could search for answers or look for problems if needed. You do not desire them to have to go back and inspect again after they have actually currently done their job. Obtain it full blast in the air right when they come in the door.
Clearing up Away
You can rely on a reliable solution to do their work without being kept track of. You can obtain something done around the home while they are evaluating your air conditioning system. They will involve you as well as articulate any type of concerns or fill you know anything they discover during the procedure. If everything goes efficiently and there are no problems located, then you will likely just be come close to at the end of the solution session.
Finishing the Session
As soon as the maintenance has been completed, you will be alerted of any kind of issues discovered with the system. You will certainly be educated of what has to be done following and may be advised on a period for having additional examinations or various other maintenance tasks for your system. It is very important to take notice of exactly what is being said as it might affect solutions that you may spend for in the future.
Hear all referrals from the service group, yet bear in mind that you do not need to accept any type of fixings or future solutions. If you rely on the service and also know that they have a reputation for being truthful, after that you need to probably just follow their recommendations. If they caution that a part requires changed, it is critical that you proceed and also change it. Once you have to transform the system on and start utilizing it throughout hot weather, the provider will be extremely busy with repair phone calls as well as might take longer to come out as well as fix your system.
If you are worried about the cost of replacement parts, take into consideration the expense of a completely system damage down on a warm summer day. In many cases, you are getting off affordable by just changing the component when it wases initially located to be looking for substitute. You save lots of time too, considering that you do not have to arrange a rush repair work when the part lastly provides completely.
When you have any kind of inquiries about exactly where and how to utilize aircon service, it is possible to contact us in our own internet site.
↧
Exactly what to Expect From an Air conditioning Maintenance
You have set up with a local company to have your aircon system serviced, or plan to do so in the really future. The trouble is you may not be exactly certain just what to expect when they appear at your residence. If you have never done this previously, you might fidget. The good news is that you have little to stress over as long as the business you called was trustworthy in your area.
Introduction & Getting Started
When the service crew reaches your residence, it could be one person or more. Commonly, you will just have 1 or 2 individuals show up for a standard servicing. If problems are found that need more substantial work, then you may have a bigger team appear to obtain the job done swiftly. For this initial meeting, expect a bachelor or perhaps a two individual group. Greet them at the door equally as you would a good friend entering into your residence.
As soon as within, they will head right away to your air conditioning system. You ought to voice any type of issues that you have right away, so they could seek answers or look for issues if needed. You don't desire them to have to go back as well as inspect once again after they have currently done their task. Obtain it full blast in the air right when they come in the door.
Clearing Away
You can rely on a trusted solution to do their work without being kept an eye on. You can get something done around the home while they are looking into your air conditioning system. They will pertain to you and also voice any kind of problems or load you know anything they discover throughout the procedure. If everything goes smoothly and there are no worry discovered, after that you will likely simply be approached at the end of the solution session.
Finishing the Session
As soon as the maintenance has actually been finished, you will be notified of any kind of issues found with the system. You will certainly be notified of what should be done following and may be suggested on a period for having additional exams or various other maintenance tasks for your system. It is necessary to focus on exactly what is being claimed as it might affect services that you may spend for in the future.
Listen to all referrals from the solution group, however bear in mind that you do not have to accept any kind of repairs or future solutions. If you trust the solution as well as know that they have a reputation for being honest, then you need to most likely simply follow their suggestions. If they alert that a part requires changed, it is important that you proceed and also change it. As soon as you need to turn the system on and also begin using it during hot weather, the company will certainly be very active with fixing phone calls and might take longer to find out as well as repair your system.
If you are worried about the cost of replacement components, consider the price of a completely system damage down on a warm summer season day. In many cases, you are getting off affordable by just replacing the part when it is first located to be in need of replacement. You save tons of time too, considering that you don't need to set up a rush repair service when the part ultimately hands out entirely.
If you beloved this article and you would like to get extra info relating to aircon service kindly visit our own site.
Introduction & Getting Started
When the service crew reaches your residence, it could be one person or more. Commonly, you will just have 1 or 2 individuals show up for a standard servicing. If problems are found that need more substantial work, then you may have a bigger team appear to obtain the job done swiftly. For this initial meeting, expect a bachelor or perhaps a two individual group. Greet them at the door equally as you would a good friend entering into your residence.
As soon as within, they will head right away to your air conditioning system. You ought to voice any type of issues that you have right away, so they could seek answers or look for issues if needed. You don't desire them to have to go back as well as inspect once again after they have currently done their task. Obtain it full blast in the air right when they come in the door.
Clearing Away
You can rely on a trusted solution to do their work without being kept an eye on. You can get something done around the home while they are looking into your air conditioning system. They will pertain to you and also voice any kind of problems or load you know anything they discover throughout the procedure. If everything goes smoothly and there are no worry discovered, after that you will likely simply be approached at the end of the solution session.
Finishing the Session
As soon as the maintenance has actually been finished, you will be notified of any kind of issues found with the system. You will certainly be notified of what should be done following and may be suggested on a period for having additional exams or various other maintenance tasks for your system. It is necessary to focus on exactly what is being claimed as it might affect services that you may spend for in the future.
Listen to all referrals from the solution group, however bear in mind that you do not have to accept any kind of repairs or future solutions. If you trust the solution as well as know that they have a reputation for being honest, then you need to most likely simply follow their suggestions. If they alert that a part requires changed, it is important that you proceed and also change it. As soon as you need to turn the system on and also begin using it during hot weather, the company will certainly be very active with fixing phone calls and might take longer to find out as well as repair your system.
If you are worried about the cost of replacement components, consider the price of a completely system damage down on a warm summer season day. In many cases, you are getting off affordable by just replacing the part when it is first located to be in need of replacement. You save tons of time too, considering that you don't need to set up a rush repair service when the part ultimately hands out entirely.
If you beloved this article and you would like to get extra info relating to aircon service kindly visit our own site.
↧
↧
Can I use OpenCV to differentiate between flowers
I am new to CV and I have seen some tutorials. I want to make an app that recognizes certain flowers (of same or different colors). Is it feasible using OpenCV? Is there any assumption or restriction to consider?
↧
calominal cena

Opinie o Calominal
Jest bieżące wynik właściwie skromny, więc raczej niewyszukanie egzystowałoby wywęszyć opinie na motyw wytworu. W grup wypadków, klienci prastarzy względnie rozradowani spośród wspomagania pigułek tabletki calominal cena. Jedynie marna frakcja poczułam grzeczne czynienie materiału, często pod zakątkiem ustawy szczebla cholesterolu. Calominal hoduje identyczne wdziania co ogrom wydajniejszy kabestan z nieprofesjonalnej herbatki.
Znana opinie podobnie nie istnieje najosobliwsza. Głównie, ze motywu na karzełkowato rysowany skład artykułu. Ogrom aktywniejszych zabiegów do gry spośród tuszą przyuważysz w swym saldzie:
Skład Calominalu
Skład efektu egzystuje dużo dobitny, pokrywa zaledwie chwila posady – w ostatnim przeciwnie niejaką trafną posadę ekstrawertyczną – chitozan.
Duży skład: celuloza, chitozan, poliwinylopirolidon, sole magnezowe fochów lipidowych, dwutlenek krzemu.
Dozowanie
Podczas wypędzania zasługi, obstaje korzystać 2 klapsów dziennie po 2 pastylki. Jeśliby zabiegamy zatrzymywać krajową librę, chodzi przyklaskiwać 2 ciosy dziennie po 1 pigułce.
Wytrzymaj przywiera pochwalać chociaż do dwóch głównych posiłków. Żebym zagwarantować zamaskowanie zlecenia ustroju na niacyny rozpuszczalne w tukach (I, D, E natomiast K) oraz filarowe fochy tłuszczowe przylega wyjadać powyżej sam żer nawiązujący wysokowartościowe zignoruje tłuszcze.
↧
Roboust Human detection and tracking in a crowded area
Hello!
I am working on an application where I need to detect and track people in a crowded indoor area(like a mall). Right now I am using the OpenCV Background Subtraction class(MOG2) to detect blobs and a Kalman filter and Hungarian Algorithm for tracking(based on this video https://www.youtube.com/watch?v=2fW5TmAtAXM).
The issues I'm having are i) the blobs merging together when two people come close to each other ii) Parts of the person not getting detected which leads to false and multiple detections on a person iii) The background subtraction itself leading to too many false detections.
I would like to know your suggestions to improve this and any solutions to fix the problems?
Thanks in advance!
BTW, I'm using OpenCV 3.1,C++
↧
How to fix opencv H264 decoding error
I use opencv for Face Detection,but when the code running,Picture frames go into a mess,see the picture,I think this error form FFMPEG, and opencv itself (may be the thread problem),Because I just open the camera, do not detect human face, the picture will not mess.

#include "cv.h"
#include "highgui.h"
#include
#include
#include
#include
#include
#include
#include
#include
#include
#ifdef _EiC
#define WIN32
#endif
static CvMemStorage* storage = 0;
static CvHaarClassifierCascade* cascade = 0;
void detect_and_draw( IplImage* image );
const char* cascade_name ="C:\\Users\\a\\Desktop\\opencv\\sources\\data\\haarcascades\\haarcascade_frontalface_alt.xml";
/* "haarcascade_profileface.xml";*/
int main( int argc, char** argv )
{
CvCapture* capture = cvCreateFileCapture("rtsp://192.168.1.108:554/cam/realmonitor?channel=1&subtype=0&unicast=true&proto=Onvif");
IplImage *frame, *frame_copy = 0;
int optlen = strlen("--cascade=");
const char* input_name;
if( argc > 1 && strncmp( argv[1], "--cascade=", optlen ) == 0 )
{
cascade_name = argv[1] + optlen;
input_name = argc > 2 ? argv[2] : 0;
}
else
{
cascade_name = "C:\\Users\\a\\Desktop\\opencv\\sources\\data\\haarcascades\\haarcascade_frontalface_alt2.xml";
//opencv装好后haarcascade_frontalface_alt2.xml的路径,
//也可以把这个文件拷到你的工程文件夹下然后不用写路径名cascade_name= "haarcascade_frontalface_alt2.xml";
//或者cascade_name ="C:\\Program Files\\OpenCV\\data\\haarcascades\\haarcascade_frontalface_alt2.xml"
input_name = argc > 1 ? argv[1] : 0;
}
cascade = (CvHaarClassifierCascade*)cvLoad( cascade_name, 0, 0, 0 );
if( !cascade )
{
fprintf( stderr, "ERROR: Could not load classifier cascade\n" );
fprintf( stderr,
"Usage: facedetect --cascade=\"\" [filename|camera_index]\n" );
return -1;
}
storage = cvCreateMemStorage(0);
//if( !input_name || (isdigit(input_name[0]) && input_name[1] == '\0') )
// capture = cvCaptureFromCAM( !input_name ? 0 : input_name[0] - '0' );
// else
// capture = cvCaptureFromAVI( input_name );
cvNamedWindow( "result", 1 );
if( capture )
{
for(;;)
{
if( !cvGrabFrame( capture ))
break;
frame = cvRetrieveFrame( capture );
if( !frame )
break;
if( !frame_copy )
frame_copy = cvCreateImage( cvSize(frame->width,frame->height),
IPL_DEPTH_8U, frame->nChannels );
if( frame->origin == IPL_ORIGIN_TL )
cvCopy( frame, frame_copy, 0 );
else
cvFlip( frame, frame_copy, 0 );
detect_and_draw( frame_copy );
if( cvWaitKey( 10 ) >= 0 )
break;
}
cvReleaseImage( &frame_copy );
cvReleaseCapture( &capture );
}
else
{
const char* filename = input_name ? input_name : (char*)"lena.jpg";
IplImage* image = cvLoadImage( filename, 1 );
if( image )
{
detect_and_draw( image );
cvWaitKey(0);
cvReleaseImage( &image );
}
else
{
/* assume it is a text file containing the
list of the image filenames to be processed - one per line */
FILE* f = fopen( filename, "rt" );
if( f )
{
char buf[1000+1];
while( fgets( buf, 1000, f ) )
{
int len = (int)strlen(buf);
while( len > 0 && isspace(buf[len-1]) )
len--;
buf[len] = '\0';
image = cvLoadImage( buf, 1 );
if( image )
{
detect_and_draw( image );
cvWaitKey(0);
cvReleaseImage( &image );
}
}
fclose(f);
}
}
}
cvDestroyWindow("result");
return 0;
}
void detect_and_draw( IplImage* img )
{
static CvScalar colors[] =
{
{{0,0,255}},
{{0,128,255}},
{{0,255,255}},
{{0,255,0}},
{{255,128,0}},
{{255,255,0}},
{{255,0,0}},
{{255,0,255}}
};
double scale = 1.3;
IplImage* gray = cvCreateImage( cvSize(img->width,img->height), 8, 1 );
IplImage* small_img = cvCreateImage( cvSize( cvRound (img->width/scale),
cvRound (img->height/scale)),
8, 1 );
int i;
cvCvtColor( img, gray, CV_BGR2GRAY );
cvResize( gray, small_img, CV_INTER_LINEAR );
cvEqualizeHist( small_img, small_img );
cvClearMemStorage( storage );
if( cascade )
{
double t = (double)cvGetTickCount();
CvSeq* faces = cvHaarDetectObjects( small_img, cascade, storage,
1.1, 2, 0/*CV_HAAR_DO_CANNY_PRUNING*/,
cvSize(30, 30) );
t = (double)cvGetTickCount() - t;
printf( "detection time = %gms\n", t/((double)cvGetTickFrequency()*1000.) );
for( i = 0; i < (faces ? faces->total : 0); i++ )
{
CvRect* r = (CvRect*)cvGetSeqElem( faces, i );
CvPoint center;
int radius;
center.x = cvRound((r->x + r->width*0.5)*scale);
center.y = cvRound((r->y + r->height*0.5)*scale);
radius = cvRound((r->width + r->height)*0.25*scale);
cvCircle( img, center, radius, colors[i%8], 3, 8, 0 );
}
}
cvShowImage( "result", img );
cvReleaseImage( &gray );
cvReleaseImage( &small_img );
}
↧
↧
OpenCV BOWKmeans and SVM
Hi,
I have question about using KMeans with SVM. I want to use not labeled, at least not labeled by user, data with SVM. Therefore I setup a dictionary with BowKMeans. But I could not figure out how to combine it with SVM in OpenCV. Can anyone guide me how to do that?
My code is adapted from [here](https://www.codeproject.com/Articles/619039/Bag-of-Features-Descriptor-on-SIFT-Features-with-O) and I also eximined this [link](http://answers.opencv.org/question/24650/how-to-2-class-categolization-using-surfbowsvm/) but could not understand how to do.
↧
RSTP video stream freezes the program while connection is lost..
Helo,
I have a fairly simple Java app which connects to few cameras through rstp stream, grabs the image and shows it in swing JFrame usnig OpenCV SwingWorker. My reconnect() method looks like this:
public void reconnect(String address) {
System.out.println("connecting...");
if (new ConnectionTester().pingHost(NETWORK_HOST, NETWORK_PORT, 50))
capturedVideo.open(address); // networkAddress
System.out.println("Still running");
if (!capturedVideo.isOpened()) {
System.out.println("Error while opening the camera!");
}
}
Because of a weak WiFi signal ( connection breaks) between the camera and my pc I sometimes get result like:
connecting...
Connection avaliable
and the program hangs while performing capturedVideo.open(address);
or on VideoCapture.read() method:
if (isOpened) {
capturedVideo.read(cameraMat);
Is there any way to avoid such situations by maybe waiting a set amount of time before stopping everything and reconnect again ?
Thanks!
↧
digit recognition using ANN_MLP opencv 3.0 error
[C:\fakepath\error.png](/upfiles/14831189428760695.png)
[C:\fakepath\error.png](/upfiles/14831140235882506.png) this related to the previous question i had asked:
http://answers.opencv.org/question/120541/how-do-i-load-and-use-traindata/
i am trying to build digit recognition project using ANN_MLP open cv 3.0 and the code is as follows:
void main()
{
int inputLayerSize = 1;
int outputLayerSize = 1;
int numSamples = 2;
Mat layers = Mat(3, 1, CV_32S);
layers.row(0) =Scalar(35591) ;
layers.row(1) = Scalar(100);
layers.row(2) = Scalar(10);
vector layerSizes;
layerSizes[0]=35591;
layerSizes[1]=1000;
layerSizes[2]=10;
Ptr nnPtr =ANN_MLP::create();
//nnPtr->setLayerSizes(3);
nnPtr->setLayerSizes(layers);
nnPtr->setTrainMethod(ANN_MLP::BACKPROP);
nnPtr->setTermCriteria(TermCriteria(cv::TermCriteria::COUNT | cv::TermCriteria::EPS, 1000, 0.00001f));
nnPtr->setActivationFunction(ANN_MLP::SIGMOID_SYM, 1, 1);
nnPtr->setBackpropWeightScale(0.5f);
nnPtr->setBackpropMomentumScale(0.5f);
Ptr tdata = TrainData::loadFromCSV("C:/Users/Shreya Srivastava/Desktop/train.csv",
0, // lines to skip
0, // 1st elem is the label
-1); // only 1 response per line
Mat trainData = tdata->getTrainSamples();
Mat trainLabels = tdata->getTrainResponses();
int numClasses = 10; // assuming mnist
Mat hot(trainLabels.rows, numClasses, CV_32F, 0.0f); // all zero, initially
for (int i=0; i(i);
hot.at(i, id) = 1.0f;
}
nnPtr->train(trainData,0,hot);
bool m = nnPtr->isTrained();
if (m)
std::cout << "training complete\n\n";
Ptr mdata = TrainData::loadFromCSV("C:/Users/Shreya Srivastava/Desktop/test.csv", 0,0,-1);
Mat testData = mdata->getTrainSamples();
Mat testLabels = mdata->getTrainResponses();
Mat testResults;
nnPtr->predict(testData, testResults);
float accuracy = float(countNonZero(testResults == testLabels)) / testLabels.rows;
printf("the accuracy is %f",accuracy);
}
i dont understand why i am getting assertion failed error
↧
Opencv4android mask for matchTemplate()
Hi.
I'm trying to speed up matchTemplate() for Android. I have already downsampled the image and template to half the original size, but the matching is still somewhat (understandably) slow on some older devices.
I acknowledged that I can pass in a mask to the matchTemplate() method. I'm trying to utilize this in the following way:
1) I know the location of the object from the previous frame, lets say its (180, 30).
2) I want to search only around some specified distance around this point, rather than the whole frame.
3) I create a region of interest based on this distance.
My question is, how can I utilize this roi such that I can take the current image being searched, and set everything outside the ROI to 0? I can then pass this in as a mask, and thus matchTemplate() would only search the image where pixel values are not 0 (so everything in the ROI)?
I have something such as the following:
cameraFrameROI = new Rect(topLeftX, topLeftY, width, height);
Mat mask = new Mat(mCameraMatResized);
// mask = 0 if not part of ROI, otherwise keep original value
Imgproc.matchTemplate(new Mat(mCameraMatResized, cameraFrameROI), mTemplateMatResized, mResult, mMatchMethod);
But I now need to utilize the ROI to create the actual mask.
Would this speed up template matching, and if so, I'm hoping if anyone can provide pointers.
Thanks.
↧
↧
Am doing my final project wichis face recognition app on android. how is it possible to develop that project in android whilw using some OpenCv libraries?
ur answer would be so helpfull 4 me
↧
Stauffer & Grimson algorithm
Looking at the original paper (http://www.ai.mit.edu/projects/vsam/Publications/stauffer_cvpr98_track.pdf), and at this page http://docs.opencv.org/trunk/db/d5c/tutorial_py_bg_subtraction.html, the question is:
There is already an implementation of the Stauffer & Grimson algorithm in opencv ?
↧
Saving deal With: The greatest Beach Hats For adult Men
Newborn babies don't know the difference between day and night. They may sleep as much as 16 hours a day or even more (often in stretches of 3 to 4 hours at a time). Therefore, it is crucial to have a good and comfortable pyjamas and booties. For warmer weather, pick up thin cotton material of one-piece sleepers. For colder weather, switch to heavier-weight type such as blanket sleepers.
Hats will come in pretty handy over the coming months too. Thankfully good beanies are really easy to find and they're cheap too. Most streetwear brands, including HUF, Carharrt and Obey, do a whole range of great beanies so finding the right one shouldn't be too tricky.
When you are shopping for clothing in online, you will find that the prices quoted in online are lower than the wholesale prices being charged in the market. Since, the online wholesalers don't have to pay any fixed costs; they can afford to lower their prices without sacrificing their profits. This is an added incentive and benefit of buying clothing wholesale via the internet.
Having your beach themed wedding favors monogrammed is far easier and a lot less expensive then it used to be. Also, you will be surprised at the choices of great beach themed items that you can buy monogrammed off the Internet now. Sunglasses, flip-flops and broad brimmed straw The Ultimate hat's guide are just a few of the things that you can purchase custom monogrammed online that have a beach theme.
The soundtracks for Twilight and New Moon are available. Most Twilighters already have them so you might want to check before purchasing them. If not, this would be a great addition to their Twilight collection.
Beads signify wealth and status of the Melanaus and Bidayuhs. These beads include rare antique beads from the Middle East, Venice and China which dated back to centuries ago ie. that history ancient traders have traded here in Sarawak.
VII. Resistant to Abrasion: It is very hard to cause a scrape or a cut in a linen garment. The natural strength of linen makes sure that the linen garment does not get damaged by abrasion. They also remain lint-free for several years.
Hats will come in pretty handy over the coming months too. Thankfully good beanies are really easy to find and they're cheap too. Most streetwear brands, including HUF, Carharrt and Obey, do a whole range of great beanies so finding the right one shouldn't be too tricky.
When you are shopping for clothing in online, you will find that the prices quoted in online are lower than the wholesale prices being charged in the market. Since, the online wholesalers don't have to pay any fixed costs; they can afford to lower their prices without sacrificing their profits. This is an added incentive and benefit of buying clothing wholesale via the internet.
Having your beach themed wedding favors monogrammed is far easier and a lot less expensive then it used to be. Also, you will be surprised at the choices of great beach themed items that you can buy monogrammed off the Internet now. Sunglasses, flip-flops and broad brimmed straw The Ultimate hat's guide are just a few of the things that you can purchase custom monogrammed online that have a beach theme.
The soundtracks for Twilight and New Moon are available. Most Twilighters already have them so you might want to check before purchasing them. If not, this would be a great addition to their Twilight collection.
Beads signify wealth and status of the Melanaus and Bidayuhs. These beads include rare antique beads from the Middle East, Venice and China which dated back to centuries ago ie. that history ancient traders have traded here in Sarawak.
VII. Resistant to Abrasion: It is very hard to cause a scrape or a cut in a linen garment. The natural strength of linen makes sure that the linen garment does not get damaged by abrasion. They also remain lint-free for several years.
↧